There are many algorithms to reduce noise in an image. A bilateral filter is often used for noise reduction while preserving edges in an image. This filter calculates a weighted average of all the pixels in the neighborhood. Also, bilateral filter takes into consideration the variation of pixel intensities for preserving edges.
OpenCV provides the bilateralFilter
function that allows to apply bilateral filter to an image. The d
parameter defines filter size. In other words, it is the diameter of each pixel neighborhood. Sigma in the color space and sigma in the coordinate space control the amount of filtering.
import cv2
img = cv2.imread('test.jpg')
grayImg = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
filteredImg = cv2.bilateralFilter(grayImg, d=5, sigmaColor=50, sigmaSpace=50)
cv2.imshow('Original image', grayImg)
cv2.imshow('Filtered image', filteredImg)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat img = imread("test.jpg");
Mat grayImg;
cvtColor(img, grayImg, COLOR_BGR2GRAY);
Mat filteredImg;
bilateralFilter(grayImg, filteredImg, 5, 50, 50);
imshow("Original image", grayImg);
imshow("Filtered image", filteredImg);
waitKey(0);
destroyAllWindows();
return 0;
}
#include <opencv2/opencv.hpp>
#include <opencv2/cudaimgproc.hpp>
using namespace cv;
int main()
{
Mat img = imread("test.jpg");
cuda::GpuMat gpuImg(img);
cuda::cvtColor(gpuImg, gpuImg, COLOR_RGB2GRAY);
Mat grayImg(gpuImg);
cuda::bilateralFilter(gpuImg, gpuImg, 5, 50, 50);
Mat filteredImg(gpuImg);
imshow("Original image", grayImg);
imshow("Filtered image", filteredImg);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat img = Imgcodecs.imread("test.jpg");
Mat grayImg = new Mat();
Imgproc.cvtColor(img, grayImg, Imgproc.COLOR_BGR2GRAY);
Mat filteredImg = new Mat();
Imgproc.bilateralFilter(grayImg, filteredImg, 5, 50, 50);
HighGui.imshow("Original image", grayImg);
HighGui.imshow("Filtered image", filteredImg);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
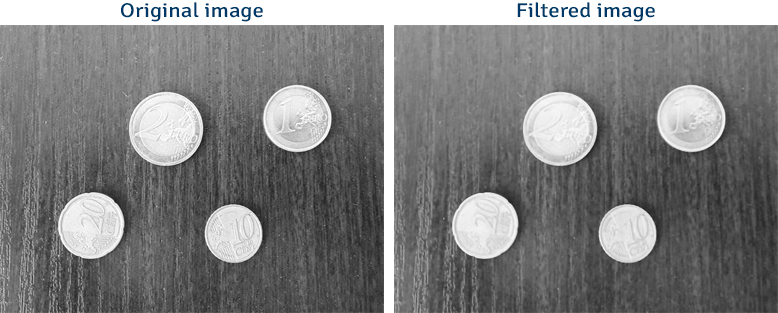
Leave a Comment
Cancel reply