Image resizing reduces the number of pixels in an image. It can be useful when want to decrease image processing time or reduce the time of training of a neural network.
OpenCV provides the resize
function which resizes the image down to or up by the specified width and height.
import cv2
width = 320
height = 240
img = cv2.imread('test.jpg')
resizedImg = cv2.resize(img, (width, height))
cv2.imshow('Original image', img)
cv2.imshow('Resized image', resizedImg)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
int width = 320;
int height = 240;
Mat img = imread("test.jpg");
Mat resizedImg;
resize(img, resizedImg, Size(width, height));
imshow("Original image", img);
imshow("Grayscale image", resizedImg);
waitKey(0);
destroyAllWindows();
return 0;
}
#include <opencv2/opencv.hpp>
#include <opencv2/cudawarping.hpp>
using namespace cv;
int main()
{
int width = 320;
int height = 240;
Mat img = imread("test.jpg");
cuda::GpuMat gpuImg(img);
cuda::resize(gpuImg, gpuImg, Size(width, height));
Mat resizedImg(gpuImg);
imshow("Original image", img);
imshow("Grayscale image", resizedImg);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.*;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
int width = 320;
int height = 240;
Mat img = Imgcodecs.imread("test.jpg");
Mat resizedImg = new Mat();
Imgproc.resize(img, resizedImg, new Size(width, height));
HighGui.imshow("Original image", img);
HighGui.imshow("Resized image", resizedImg);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
Result:
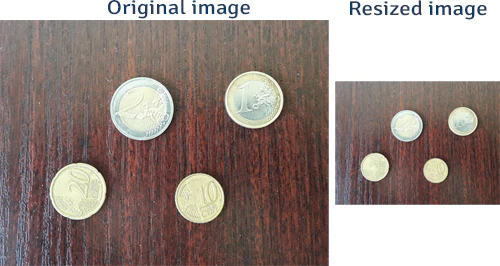
Leave a Comment
Cancel reply