Object detection based on Haar cascades is a machine learning technique where a cascade function is trained using positive and negative images. It is used to detect objects in an image, such as faces.
Download image for testing:
curl -o test.jpg https://raw.githubusercontent.com/ageitgey/face_recognition/master/examples/two_people.jpg
Download pretrained Haar cascade model:
curl -o haarcascade_frontalface_alt.xml https://raw.githubusercontent.com/opencv/opencv/master/data/haarcascades/haarcascade_frontalface_alt.xml
Note: Using CUDA, Haar cascade model structure is different. In that case, download the following file instead of the previous one:
curl -o haarcascade_frontalface_alt.xml https://raw.githubusercontent.com/opencv/opencv/master/data/haarcascades_cuda/haarcascade_frontalface_alt.xml
CascadeClassifier
is created, and Haar cascade model is loaded from an XML file. The detectMultiScale
method is used to detect faces in an image. The method returns boundary rectangles for the detected faces which displayed on the image.
import cv2
haarCascade = 'haarcascade_frontalface_alt.xml'
img = cv2.imread('test.jpg')
grayImg = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
classifier = cv2.CascadeClassifier(haarCascade)
faces = classifier.detectMultiScale(grayImg)
for x, y, w, h in faces:
cv2.rectangle(img, (x, y), (x + w, y + h), color=(0, 255, 0), thickness=2)
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
std::string haarCascade = "haarcascade_frontalface_alt.xml";
Mat img = imread("test.jpg");
Mat grayImg;
cvtColor(img, grayImg, COLOR_BGR2GRAY);
CascadeClassifier classifier(haarCascade);
std::vector<Rect> faces;
classifier.detectMultiScale(grayImg, faces);
for (int i = 0; i < faces.size(); i++) {
rectangle(img, faces[i], Scalar(0, 255, 0), 2);
}
imshow("Image", img);
waitKey(0);
destroyAllWindows();
return 0;
}
#include <opencv2/opencv.hpp>
#include <opencv2/cudaimgproc.hpp>
#include <opencv2/cudaobjdetect.hpp>
using namespace cv;
int main()
{
std::string haarCascade = "haarcascade_frontalface_alt.xml";
Mat img = imread("test.jpg");
cuda::GpuMat gpuImg(img);
cuda::cvtColor(gpuImg, gpuImg, COLOR_RGB2GRAY);
Ptr<cuda::CascadeClassifier> classifier = cuda::CascadeClassifier::create(haarCascade);
cuda::GpuMat gpuFaces;
classifier->detectMultiScale(gpuImg, gpuFaces);
std::vector<Rect> faces;
classifier->convert(gpuFaces, faces);
for (int i = 0; i < faces.size(); i++) {
rectangle(img, faces[i], Scalar(0, 255, 0), 2);
}
imshow("Image", img);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.*;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
import org.opencv.objdetect.CascadeClassifier;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
String haarCascade = "haarcascade_frontalface_alt.xml";
Mat img = Imgcodecs.imread("test.jpg");
Mat grayImg = new Mat();
Imgproc.cvtColor(img, grayImg, Imgproc.COLOR_BGR2GRAY);
CascadeClassifier classifier = new CascadeClassifier(haarCascade);
MatOfRect faces = new MatOfRect();
classifier.detectMultiScale(grayImg, faces);
for (int i = 0; i < faces.rows(); i++) {
Rect face = new Rect(faces.get(i, 0));
Imgproc.rectangle(img, face, new Scalar(0, 255, 0), 2);
}
HighGui.imshow("Image", img);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
Result:
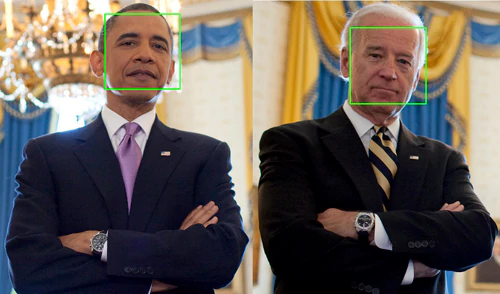
The detectMultiScale
method accepts two parameters, which can be adjusted if the method detects faces incorrectly or no faces are detected at all.
scaleFactor
- defines how much the image size should be reduced at each image scale. Default value is1.1
.minNeighbors
- defines how many neighbor pixels each candidate rectangle should have to be considered a face. Default value is3
. Higher value results in fewer detections, but with higher quality. Recommended values are 3, 4, 5, or 6.
Leave a Comment
Cancel reply