OpenCV has many functions to draw various shapes such as line, rectangle, circle, etc.
The arrowedLine
function draws an arrow line that pointing from the first point to the second one by specifying x and y coordinates for each point.
import cv2
import numpy as np
img = np.zeros((100, 300, 3), dtype=np.uint8)
x1, y1 = 100, 20
x2, y2 = 200, 70
color = (0, 255, 0)
thickness = 2
cv2.arrowedLine(img, (x1, y1), (x2, y2), color, thickness)
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat img = Mat::zeros(100, 300, CV_8UC3);
int x1 = 100, y1 = 20;
int x2 = 200, y2 = 70;
Scalar color(0, 255, 0);
int thickness = 2;
arrowedLine(img, Point(x1, y1), Point(x2, y2), color, thickness);
imshow("Image", img);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.*;
import org.opencv.highgui.HighGui;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat img = Mat.zeros(100, 300, CvType.CV_8UC3);
int x1 = 100, y1 = 20;
int x2 = 200, y2 = 70;
Scalar color = new Scalar(0, 255, 0);
int thickness = 2;
Imgproc.arrowedLine(img, new Point(x1, y1), new Point(x2, y2), color, thickness);
HighGui.imshow("Image", img);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
Result:
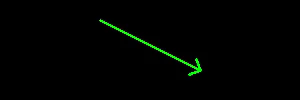
Leave a Comment
Cancel reply