OpenCV offers many drawing functions that can be used to draw geometric shapes and write text on an image.
The putText
function can be used to write text on an image by specifying x and y coordinates, font family, font scale and other parameters.
import cv2
import numpy as np
img = np.zeros((100, 300, 3), dtype=np.uint8)
x, y = 120, 50
font = cv2.FONT_HERSHEY_SIMPLEX
fontScale = 1.0
color = (0, 255, 0)
thickness = 2
cv2.putText(img, 'Test', (x, y), font, fontScale, color, thickness)
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat img = Mat::zeros(100, 300, CV_8UC3);
int x = 120, y = 50;
int font = FONT_HERSHEY_SIMPLEX;
double fontScale = 1.0;
Scalar color(0, 255, 0);
int thickness = 2;
putText(img, "Test", Point(x, y), font, fontScale, color, thickness);
imshow("Image", img);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.*;
import org.opencv.highgui.HighGui;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat img = Mat.zeros(100, 300, CvType.CV_8UC3);
int x = 120, y = 50;
int font = Imgproc.FONT_HERSHEY_SIMPLEX;
double fontScale = 1.0;
Scalar color = new Scalar(0, 255, 0);
int thickness = 2;
Imgproc.putText(img, "Test", new Point(x, y), font, fontScale, color, thickness);
HighGui.imshow("Image", img);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
Result:
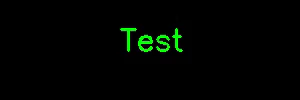
Leave a Comment
Cancel reply