OpenCV provides many functions to draw geometric shapes such as line, rectangle, circle, etc.
The ellipse
function can be used to draw ellipse or ellipse arc by providing x and y center coordinates, axes length, angle and other parameters.
import cv2
import numpy as np
img = np.zeros((100, 300, 3), dtype=np.uint8)
center = (150, 50)
axes = (60, 30)
angle = 0
startAngle = 0
endAngle = 360
color = (0, 255, 0)
thickness = 2
cv2.ellipse(img, center, axes, angle, startAngle, endAngle, color, thickness)
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat img = Mat::zeros(100, 300, CV_8UC3);
Point center(150, 50);
Size axes(60, 30);
int angle = 0;
int startAngle = 0;
int endAngle = 360;
Scalar color(0, 255, 0);
int thickness = 2;
ellipse(img, center, axes, angle, startAngle, endAngle, color, thickness);
imshow("Image", img);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.*;
import org.opencv.highgui.HighGui;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat img = Mat.zeros(100, 300, CvType.CV_8UC3);
Point center = new Point(150, 50);
Size axes = new Size(60, 30);
int angle = 0;
int startAngle = 0;
int endAngle = 360;
Scalar color = new Scalar(0, 255, 0);
int thickness = 2;
Imgproc.ellipse(img, center, axes, angle, startAngle, endAngle, color, thickness);
HighGui.imshow("Image", img);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
Result:
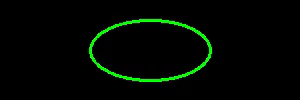
Leave a Comment
Cancel reply