During image processing, RGB images are often converted to grayscale images because smaller amount of data allows performing more complex image processing operations faster.
This tutorial provides example how to convert RGB image to grayscale image using OpenCV.
OpenCV provides the cvtColor
function that allows to convert an image from one color space to another. This function accepts color conversion code. BGR2GRAY
code is used to convert RGB image to grayscale image. Note that, OpenCV loads an image where the order of the color channels is Blue, Green, Red (BGR) instead of RGB.
OpenCV uses weighted method, also known as luminosity method, for RGB to grayscale conversion. The grayscale value is calculated as the weighted sum of the R, G, and B color components. The formula is:
import cv2
img = cv2.imread('test.jpg')
grayImg = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
cv2.imshow('RGB image', img)
cv2.imshow('Grayscale image', grayImg)
cv2.waitKey(0)
cv2.destroyAllWindows()
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat img = imread("test.jpg");
Mat grayImg;
cvtColor(img, grayImg, COLOR_BGR2GRAY);
imshow("RGB image", img);
imshow("Grayscale image", grayImg);
waitKey(0);
destroyAllWindows();
return 0;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
#include <opencv2/opencv.hpp>
#include <opencv2/cudaimgproc.hpp>
using namespace cv;
int main()
{
Mat img = imread("test.jpg");
cuda::GpuMat gpuImg(img);
cuda::cvtColor(gpuImg, gpuImg, COLOR_RGB2GRAY);
Mat grayImg(gpuImg);
imshow("RGB image", img);
imshow("Grayscale image", grayImg);
waitKey(0);
destroyAllWindows();
return 0;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
package app;
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat img = Imgcodecs.imread("test.jpg");
Mat grayImg = new Mat();
Imgproc.cvtColor(img, grayImg, Imgproc.COLOR_BGR2GRAY);
HighGui.imshow("RGB image", img);
HighGui.imshow("Grayscale image", grayImg);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
Result:
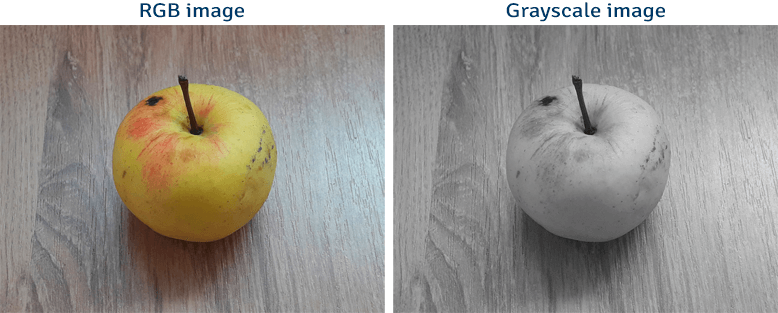
Leave a Comment
Cancel reply