Image thresholding is a technique that allows to perform image binarization based on pixel values. Usually, if the pixel value is greater than a threshold, it is set to a maximum value (often 255 - white), otherwise it is set to 0 (black). There are various methods to calculate threshold value. One of them is Otsu's method. It is a global thresholding algorithm, where a single threshold value is applied for the whole image.
OpenCV offers the threshold
function to perform image thresholding. We can specify THRESH_OTSU
flag as argument to apply Otsu's method to calculate threshold value.
import cv2
img = cv2.imread('test.jpg')
grayImg = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
thresh, binaryImg = cv2.threshold(grayImg, 0, 255, cv2.THRESH_OTSU)
print(thresh)
cv2.imshow('Grayscale image', grayImg)
cv2.imshow('Binary image', binaryImg)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <iostream>
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat img = imread("test.jpg");
Mat grayImg;
cvtColor(img, grayImg, COLOR_BGR2GRAY);
Mat binaryImg;
double thresh = threshold(grayImg, binaryImg, 0, 255, THRESH_OTSU);
std::cout << thresh << std::endl;
imshow("Grayscale image", grayImg);
imshow("Binary image", binaryImg);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat img = Imgcodecs.imread("test.jpg");
Mat grayImg = new Mat();
Imgproc.cvtColor(img, grayImg, Imgproc.COLOR_BGR2GRAY);
Mat binaryImg = new Mat();
double thresh = Imgproc.threshold(grayImg, binaryImg, 0, 255, Imgproc.THRESH_OTSU);
System.out.println(thresh);
HighGui.imshow("Grayscale image", grayImg);
HighGui.imshow("Binary image", binaryImg);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
Calculated threshold value (T) is 155. Result:
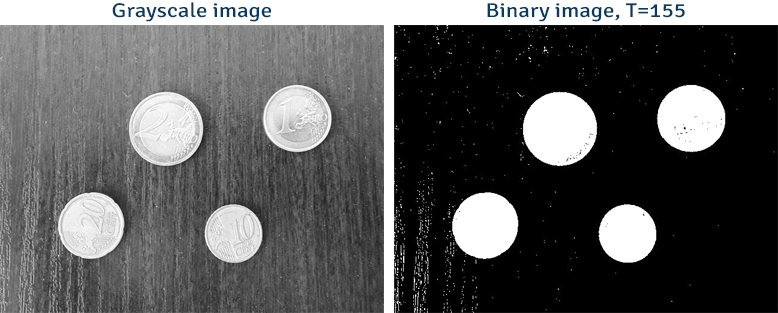
The 2 Comments Found
What is "T" in output image
Hi,
T=155 refers to a threshold value for an image, which is automatically determined when using Otsu's method.
Leave a Comment
Cancel reply