Erosion is a morphological image processing operation that removes the boundaries of the foreground object. In most case, foreground pixels are white. To apply an erosion operation to an image, the kernel (structuring element) is defined. The kernel goes through the image from left-to-right and top-to-bottom. A foreground pixel in the input image will be kept if all the pixels inside the kernel are 1. Otherwise, the pixels are set to 0. Often, erosion operation is used to remove small objects or isolate connected objects in an image.
OpenCV provides the erode
function to apply erosion operation to an image.
import cv2
import numpy as np
inputImg = cv2.imread('test.jpg')
inputImg = cv2.cvtColor(inputImg, cv2.COLOR_BGR2GRAY)
_, inputImg = cv2.threshold(inputImg, 0, 255, cv2.THRESH_OTSU)
kernel = np.ones((4, 4))
outputImg = cv2.erode(inputImg, kernel)
cv2.imshow('Input image', inputImg)
cv2.imshow('Output image', outputImg)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat inputImg = imread("test.jpg");
cvtColor(inputImg, inputImg, COLOR_BGR2GRAY);
threshold(inputImg, inputImg, 0, 255, THRESH_OTSU);
Mat kernel = getStructuringElement(MORPH_RECT, Size(4, 4));
Mat outputImg;
erode(inputImg, outputImg, kernel);
imshow("Input image", inputImg);
imshow("Output image", outputImg);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.*;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat inputImg = Imgcodecs.imread("test.jpg");
Imgproc.cvtColor(inputImg, inputImg, Imgproc.COLOR_BGR2GRAY);
Imgproc.threshold(inputImg, inputImg, 0, 255, Imgproc.THRESH_OTSU);
Mat kernel = Imgproc.getStructuringElement(Imgproc.MORPH_RECT, new Size(4, 4));
Mat outputImg = new Mat();
Imgproc.erode(inputImg, outputImg, kernel);
HighGui.imshow("Input image", inputImg);
HighGui.imshow("Output image", outputImg);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
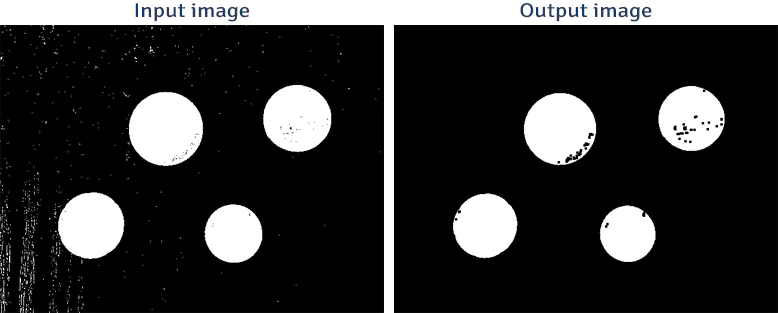
Leave a Comment
Cancel reply