Gaussian blurring is an image smoothing algorithm that used to remove noise from the image.
OpenCV has GaussianBlur
function that allows to apply Gaussian blurring on image. Smoothing is controlled by using 3 parameters, such as kernel size (ksize
) and standard deviation in the X (sigmaX
) and Y (sigmaY
) directions. If only sigmaX
is set, then sigmaY
will be the same as sigmaX
. If both parameters sigmaX
and sigmaY
are set to zeros, then they are calculated from the kernel size.
The following code applies Gaussian blurring on an image using 7x7 kernel and sigmaX
equal to 0. Note that sigmaY
is automatically set to 0 as well.
import cv2
inputImg = cv2.imread('test.jpg')
inputImg = cv2.cvtColor(inputImg, cv2.COLOR_BGR2GRAY)
outputImg = cv2.GaussianBlur(inputImg, (7, 7), 0)
cv2.imshow('Input image', inputImg)
cv2.imshow('Output image', outputImg)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat inputImg = imread("test.jpg");
cvtColor(inputImg, inputImg, COLOR_BGR2GRAY);
Mat outputImg;
GaussianBlur(inputImg, outputImg, Size(7, 7), 0);
imshow("Input image", inputImg);
imshow("Output image", outputImg);
waitKey(0);
destroyAllWindows();
return 0;
}
#include <opencv2/opencv.hpp>
#include <opencv2/cudaimgproc.hpp>
#include <opencv2/cudafilters.hpp>
using namespace cv;
int main()
{
Mat img = imread("test.jpg");
cuda::GpuMat gpuInputImg(img), gpuOutpuImg;
cuda::cvtColor(gpuInputImg, gpuInputImg, COLOR_RGB2GRAY);
Mat inputImg(gpuInputImg);
Ptr<cuda::Filter> filter = cuda::createGaussianFilter(gpuInputImg.type(), gpuOutpuImg.type(), Size(7, 7), 0);
filter->apply(gpuInputImg, gpuOutpuImg);
Mat outputImg(gpuOutpuImg);
imshow("Input image", inputImg);
imshow("Output image", outputImg);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.*;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat inputImg = Imgcodecs.imread("test.jpg");
Imgproc.cvtColor(inputImg, inputImg, Imgproc.COLOR_BGR2GRAY);
Mat outputImg = new Mat();
Imgproc.GaussianBlur(inputImg, outputImg, new Size(7, 7), 0);
HighGui.imshow("Input image", inputImg);
HighGui.imshow("Output image", outputImg);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
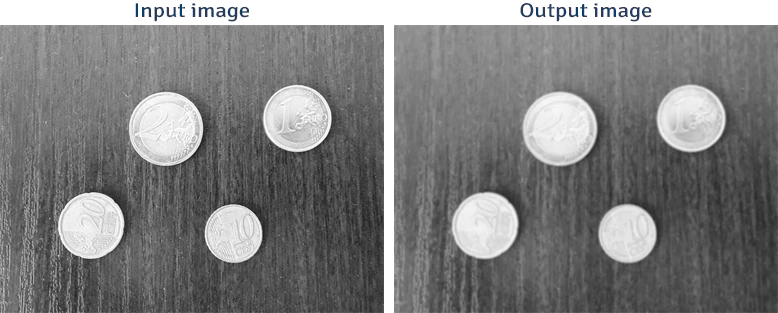
Leave a Comment
Cancel reply