Canny edge detection is an image processing algorithm that allows to detect edges in an image. It is a multi-stage algorithm that involves a series of steps such as noise reduction, find the intensity gradient of the image, non-maximum suppression and hysteresis thresholding.
OpenCV provides the Canny
function that allows to find edges in an image using Canny algorithm. Function accepts first and second threshold that used in hysteresis procedure.
import cv2
inputImg = cv2.imread('test.jpg')
inputImg = cv2.cvtColor(inputImg, cv2.COLOR_BGR2GRAY)
outputImg = cv2.Canny(inputImg, 400, 500)
cv2.imshow('Input image', inputImg)
cv2.imshow('Output image', outputImg)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat inputImg = imread("test.jpg");
cvtColor(inputImg, inputImg, COLOR_BGR2GRAY);
Mat outputImg;
Canny(inputImg, outputImg, 400, 500);
imshow("Input image", inputImg);
imshow("Output image", outputImg);
waitKey(0);
destroyAllWindows();
return 0;
}
#include <opencv2/opencv.hpp>
#include <opencv2/cudaimgproc.hpp>
using namespace cv;
int main()
{
Mat img = imread("test.jpg");
cuda::GpuMat gpuInputImg(img), gpuOutpuImg;
cuda::cvtColor(gpuInputImg, gpuInputImg, COLOR_RGB2GRAY);
Mat inputImg(gpuInputImg);
Ptr<cuda::CannyEdgeDetector> canny = cuda::createCannyEdgeDetector(400, 500);
canny->detect(gpuInputImg, gpuOutpuImg);
Mat outputImg(gpuOutpuImg);
imshow("Input image", inputImg);
imshow("Output image", outputImg);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.*;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat inputImg = Imgcodecs.imread("test.jpg");
Imgproc.cvtColor(inputImg, inputImg, Imgproc.COLOR_BGR2GRAY);
Mat outputImg = new Mat();
Imgproc.Canny(inputImg, outputImg, 400, 500);
HighGui.imshow("Input image", inputImg);
HighGui.imshow("Output image", outputImg);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
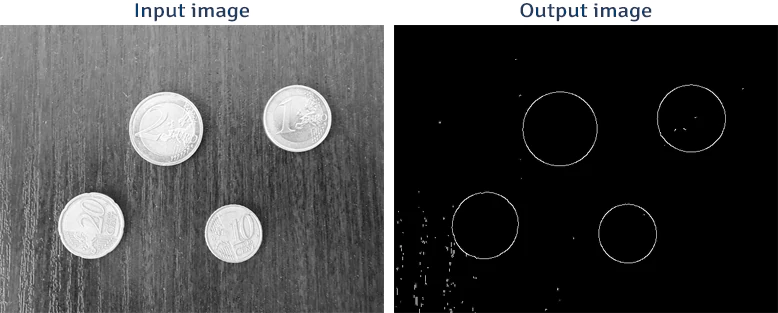
Leave a Comment
Cancel reply