HSV is a color space that has three components: hue, saturation and value. When implementing object tracking based on color, images are often converted from RGB to HSV color space. Using HSV is much easier to represent a color and extract a colored object from an image than using RGB color space.
This tutorial provides an example how to convert an image from RGB to HSV color space using OpenCV.
OpenCV has the cvtColor
function which is used for converting an image from one color space to another. This function accepts color conversion code. COLOR_BGR2HSV
code allows converting from RGB to HSV color space. Note that, OpenCV loads an image where the order of the color channels is Blue, Green, Red (BGR) instead of RGB.
import cv2
img = cv2.imread('test.jpg')
hsvImg = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
cv2.imshow('RGB image', img)
cv2.imshow('HSV image', hsvImg)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat img = imread("test.jpg");
Mat hsvImg;
cvtColor(img, hsvImg, COLOR_BGR2HSV);
imshow("RGB image", img);
imshow("HSV image", hsvImg);
waitKey(0);
destroyAllWindows();
return 0;
}
#include <opencv2/opencv.hpp>
#include <opencv2/cudaimgproc.hpp>
using namespace cv;
int main()
{
Mat img = imread("test.jpg");
cuda::GpuMat gpuImg(img);
cuda::cvtColor(gpuImg, gpuImg, COLOR_BGR2HSV);
Mat hsvImg(gpuImg);
imshow("RGB image", img);
imshow("HSV image", hsvImg);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat img = Imgcodecs.imread("test.jpg");
Mat hsvImg = new Mat();
Imgproc.cvtColor(img, hsvImg, Imgproc.COLOR_BGR2HSV);
HighGui.imshow("RGB image", img);
HighGui.imshow("HSV image", hsvImg);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
Result:
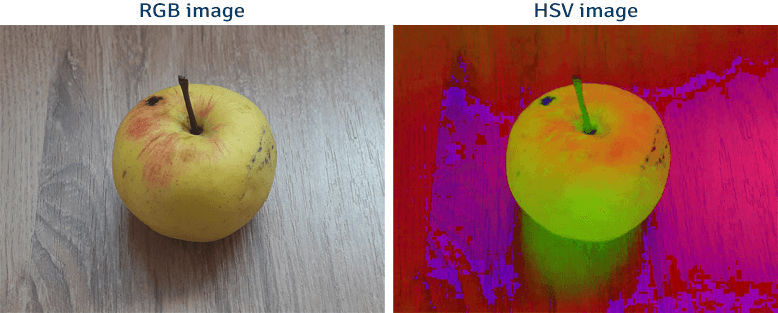
Leave a Comment
Cancel reply