OpenCV provides functionality to draw geometric shapes such as line, rectangle, circle, etc.
The polylines
function can be used for drawing polyline. It is a continuous line composed of one or more line segments.
import cv2
import numpy as np
img = np.zeros((100, 300, 3), dtype=np.uint8)
points = np.array([[100, 60], [140, 20], [180, 20], [220, 60]])
isClosed = True
color = (0, 255, 0)
thickness = 2
cv2.polylines(img, [points], isClosed, color, thickness)
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat img = Mat::zeros(100, 300, CV_8UC3);
std::vector<std::vector<Point>> points = {
{Point(100, 60), Point(140, 20), Point(180, 20), Point(220, 60)}
};
bool isClosed = true;
Scalar color(0, 255, 0);
int thickness = 2;
polylines(img, points, isClosed, color, thickness);
imshow("Image", img);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.*;
import org.opencv.highgui.HighGui;
import org.opencv.imgproc.Imgproc;
import java.util.ArrayList;
import java.util.List;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat img = Mat.zeros(100, 300, CvType.CV_8UC3);
List<MatOfPoint> points = new ArrayList<>();
points.add(
new MatOfPoint(
new Point(100, 60), new Point(140, 20),
new Point(180, 20), new Point(220, 60)
)
);
boolean isClosed = true;
Scalar color = new Scalar(0, 255, 0);
int thickness = 2;
Imgproc.polylines(img, points, isClosed, color, thickness);
HighGui.imshow("Image", img);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
Result:
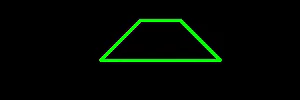
Leave a Comment
Cancel reply