OpenCV provides various functions for drawing geometric shapes such as line, rectangle, circle, etc.
The drawMarker
function draws marker on an image by predefined x and y coordinates, marker type, size and other parameters.
import cv2
import numpy as np
img = np.zeros((100, 300, 3), dtype=np.uint8)
x, y = 150, 50
color = (0, 255, 0)
markerType = cv2.MARKER_CROSS
markerSize = 15
thickness = 2
cv2.drawMarker(img, (x, y), color, markerType, markerSize, thickness)
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat img = Mat::zeros(100, 300, CV_8UC3);
int x = 150, y = 50;
Scalar color(0, 255, 0);
int markerType = MARKER_CROSS;
int markerSize = 15;
int thickness = 2;
drawMarker(img, Point(x, y), color, markerType, markerSize, thickness);
imshow("Image", img);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.*;
import org.opencv.highgui.HighGui;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat img = Mat.zeros(100, 300, CvType.CV_8UC3);
int x = 150, y = 50;
Scalar color = new Scalar(0, 255, 0);
int markerType = Imgproc.MARKER_CROSS;
int markerSize = 15;
int thickness = 2;
Imgproc.drawMarker(img, new Point(x, y), color, markerType, markerSize, thickness);
HighGui.imshow("Image", img);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
Result:
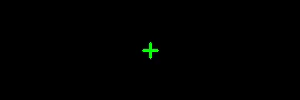
Leave a Comment
Cancel reply