An RGB image has three channels: red, green and blue. When working with image preprocessing in computer vision applications, may need to extract individual channels such as red, green and blue from an RGB image. This tutorial demonstrates how to do that using OpenCV.
import cv2
img = cv2.imread('test.jpg')
blueChannel = img[:, :, 0]
greenChannel = img[:, :, 1]
redChannel = img[:, :, 2]
cv2.imshow('Original image', img)
cv2.imshow('Blue channel', blueChannel)
cv2.imshow('Green channel', greenChannel)
cv2.imshow('Red channel', redChannel)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat img = imread("test.jpg");
Mat blueChannel, greenChannel, redChannel;
extractChannel(img, blueChannel, 0);
extractChannel(img, greenChannel, 1);
extractChannel(img, redChannel, 2);
imshow("RGB image", img);
imshow("Blue channel", blueChannel);
imshow("Green channel", greenChannel);
imshow("Red channel", redChannel);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.*;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat img = Imgcodecs.imread("test.jpg");
Mat blueChannel = new Mat(), greenChannel = new Mat(), redChannel = new Mat();
Core.extractChannel(img, blueChannel, 0);
Core.extractChannel(img, greenChannel, 1);
Core.extractChannel(img, redChannel, 2);
HighGui.imshow("RGB image", img);
HighGui.imshow("Blue channel", blueChannel);
HighGui.imshow("Green channel", greenChannel);
HighGui.imshow("Red channel", redChannel);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
Result:
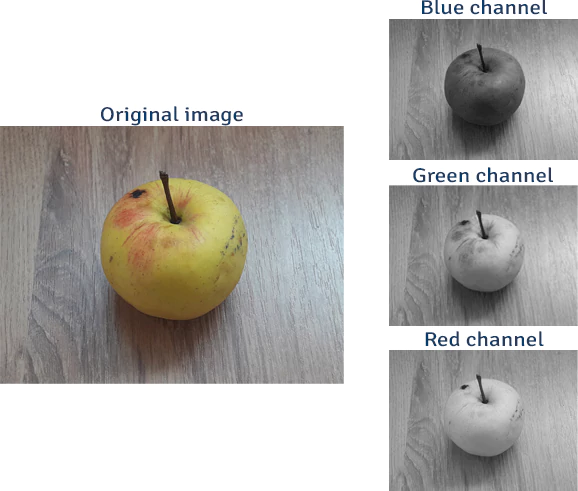
Leave a Comment
Cancel reply