There might be a case to add a custom HTTP header to all the responses in Symfony application. It can be achieved by adding header on each controller method. However, a better solution is to add header globally to all the responses.
This tutorial provides example how to add custom header to every response in Symfony 7 application.
We can create event subscriber which listens kernel.response
event. This event is dispatched when the Response
object is returned by the controller or kernel.view
listener. It can be useful for adding or modifying HTTP headers in the response before sending it back to the client.
src/EventSubscriber/ResponseSubscriber.php
<?php
namespace App\EventSubscriber;
use Symfony\Component\EventDispatcher\EventSubscriberInterface;
use Symfony\Component\HttpKernel\Event\ResponseEvent;
class ResponseSubscriber implements EventSubscriberInterface
{
public static function getSubscribedEvents(): array
{
return [ResponseEvent::class => 'onKernelResponse'];
}
public function onKernelResponse(ResponseEvent $event): void
{
if (!$event->isMainRequest()) {
return;
}
$response = $event->getResponse();
$response->headers->set('X-App-Message', 'Hello world');
}
}
You can verify response headers using web browser:
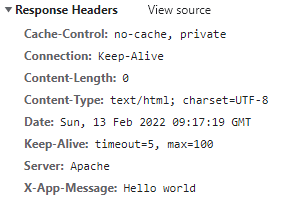
Leave a Comment
Cancel reply