Images can look either too light or too dark. Gamma correction is a method that allows to control the brightness of an image. The formula used to get a gamma corrected image is given below:
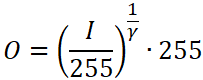
I
- input pixel value [0, 255].O
- output pixel value [0, 255].γ
- gamma that controls image brightness. If gamma < 1 then the image will be darker, if gamma > 1 then the image will be lighter. A gamma = 1 has no effect.
An image pixel values are converted from the range [0, 255] to [0, 1.0]. After calculations, values are converted back to the range [0, 255].
Gamma correction can be implemented using lookup table (LUT). It maps the input pixel values to the output values. For each pixel value in the range [0, 255] is calculated a corresponding gamma corrected value. OpenCV provides the LUT
function which performs a lookup table transform.
import cv2
import numpy as np
def gammaCorrection(src, gamma):
invGamma = 1 / gamma
table = [((i / 255) ** invGamma) * 255 for i in range(256)]
table = np.array(table, np.uint8)
return cv2.LUT(src, table)
img = cv2.imread('test.jpg')
gammaImg = gammaCorrection(img, 2.2)
cv2.imshow('Original image', img)
cv2.imshow('Gamma corrected image', gammaImg)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
void gammaCorrection(const Mat &src, Mat &dst, const float gamma)
{
float invGamma = 1 / gamma;
Mat table(1, 256, CV_8U);
uchar *p = table.ptr();
for (int i = 0; i < 256; ++i) {
p[i] = (uchar) (pow(i / 255.0, invGamma) * 255);
}
LUT(src, table, dst);
}
int main()
{
Mat img = imread("test.jpg");
Mat gammaImg;
gammaCorrection(img, gammaImg, 2.2);
imshow("Original image", img);
imshow("Gamma corrected image", gammaImg);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.Core;
import org.opencv.core.CvType;
import org.opencv.core.Mat;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void gammaCorrection(Mat src, Mat dst, float gamma)
{
float invGamma = 1 / gamma;
Mat table = new Mat(1, 256, CvType.CV_8U);
for (int i = 0; i < 256; ++i) {
table.put(0, i, (int) (Math.pow(i / 255.0f, invGamma) * 255));
}
Core.LUT(src, table, dst);
}
public static void main(String[] args)
{
Mat img = Imgcodecs.imread("test.jpg");
Mat gammaImg = new Mat();
gammaCorrection(img, gammaImg, 2.2f);
HighGui.imshow("Original image", img);
HighGui.imshow("Gamma corrected image", gammaImg);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
Result:
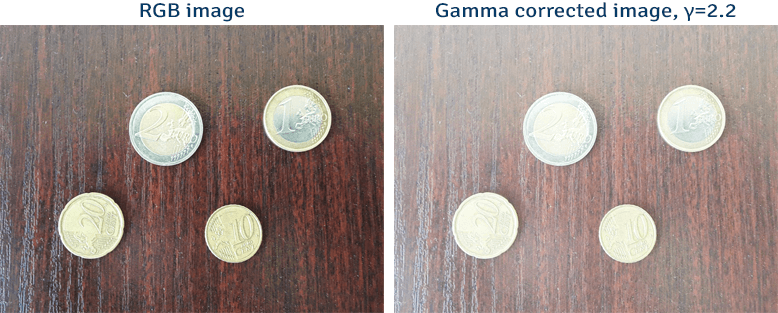
The 2 Comments Found
Is there any method through which we know the right gamma value for the image.
Hi,
Determining the right gamma value for an image often involves experimentation and visual assessment. In this process, try different gamma values and measure your system accuracy.
Leave a Comment
Cancel reply