Qt, a powerful C++ framework, provides a user-friendly way to create cross-platform applications with a graphical user interface. One common requirement in applications is to allow users to choose a file using a file dialog, and then display the selected file's path for further processing. This tutorial explains how to display file path after choosing file from dialog in Qt 6.
The provided code creates a simple Qt application which consists of a window containing a horizontal layout, which includes a button and label. When the button is clicked, a file dialog is shown, allowing the user to select a file. If a file is chosen, the label's text is updated to include the selected file's path.
#include <QApplication>
#include <QHBoxLayout>
#include <QLabel>
#include <QPushButton>
#include <QFileDialog>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
auto *window = new QWidget();
QHBoxLayout layout(window);
QPushButton button("Choose File", window);
QLabel label("File Path:", window);
QObject::connect(&button, &QPushButton::clicked, [&]() {
QString filePath = QFileDialog::getOpenFileName(window, "Choose File");
if (!filePath.isEmpty()) {
label.setText("File Path: " + filePath);
}
});
layout.addWidget(&button);
layout.addWidget(&label);
window->setLayout(&layout);
window->show();
return QApplication::exec();
}
Here's how the application looks:
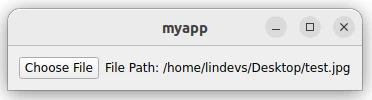
Leave a Comment
Cancel reply