In graphical user interface (GUI) applications, displaying real-time information is a common requirement. One fundamental element is the display of constantly updated time. Whether it's a digital clock in the application or a timestamp reflecting the current time, Qt provides a straightforward and efficient way to achieve this. This tutorial explains how to display constantly updated time in Qt 6.
In the main
function, a QLabel
is created and configured to show the time. The displayTime
function is initially called to set the initial time. A QTimer
is set up to trigger the displayTime
function every second, ensuring the clock updates continuously. The current time is displayed in the hh:mm:ss
format (e.g. 14:50:30).
#include <QApplication>
#include <QLabel>
#include <QTimer>
#include <QTime>
void displayTime(QLabel *label)
{
label->setText(QTime::currentTime().toString("hh:mm:ss"));
}
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
auto *label = new QLabel();
label->setAlignment(Qt::AlignCenter);
label->resize(200, 100);
displayTime(label);
label->show();
QTimer timer;
QObject::connect(&timer, &QTimer::timeout, [&label]() { displayTime(label); });
timer.start(1000);
return QApplication::exec();
}
Here's how the application looks:
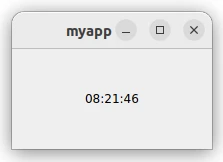
Leave a Comment
Cancel reply