GitHub provides API that allows to manage repositories and get details of them, manage pull requests and issues, get a list of stargazers and watchers, etc.
This tutorial provides example how to get the stargazers count of GitHub repository using ESP8266 NodeMCU development board.
Components
No. | Component | Quantity |
---|---|---|
1. | ESP8266 NodeMCU + Micro USB cable | 1 |
Library
For JSON data parsing we will use ArduinoJson library. It can be installed using PlatformIO from the command line.
pio project init --board nodemcuv2
pio lib install "bblanchon/ArduinoJson"
Project configuration file:
platformio.ini
[env:nodemcuv2]
platform = espressif8266
board = nodemcuv2
framework = arduino
lib_deps = bblanchon/ArduinoJson@^6.17
GitHub API integration
In order to use the GitHub API we need to create token. Follow these steps to do this:
- Sign in to GitHub and go to settings page to manage personal access tokens.
- Click "Generate new token".
- In the new page provide a note and then click "Generate token". After that you will be redirected back to settings page.
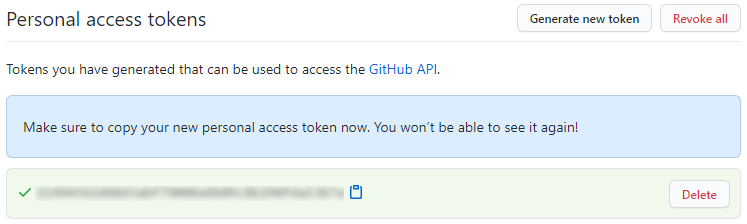
- Copy an access token. It will be used to get the stargazers count of GitHub repository.
Code
Wi-Fi network credentials (SSID and password), GitHub API URL, API endpoint and token are stored as a constants. API endpoint to get a repository details is defined as follows:
/repos/{owner}/{repo}
In the setup
function, serial communication is initialized and connection is established to a Wi-Fi network. To reduce memory consumption we use filtering to leave only required parameter in JSON document.
In the loop
function, we send GET request to a server to retrieve a repository details. A token is provided in the Authorization
header. The response is returned in JSON format. We deserialize a JSON data and print the stargazers count to the serial port.
src/main.cpp
#include <Arduino.h>
#include <ESP8266WiFi.h>
#include <ESP8266HTTPClient.h>
#include <ArduinoJson.h>
const char *WIFI_SSID = "YOUR WIFI NETWORK NAME";
const char *WIFI_PASSWORD = "YOUR WIFI PASSWORD";
const String ENDPOINT = "/repos/esp8266/Arduino"; // /repos/{owner}/{repo}
const String URL = "https://api.github.com" + ENDPOINT;
const String TOKEN = "YOUR GITHUB API TOKEN";
WiFiClientSecure client;
HTTPClient httpsClient;
StaticJsonDocument<16> filter;
StaticJsonDocument<64> doc;
void setup()
{
Serial.begin(9600);
WiFi.mode(WIFI_STA);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("Connected");
filter["stargazers_count"] = true;
client.setInsecure();
}
void loop()
{
httpsClient.begin(client, URL);
httpsClient.addHeader("Authorization", "token " + TOKEN);
httpsClient.GET();
deserializeJson(doc, httpsClient.getStream(), DeserializationOption::Filter(filter));
httpsClient.end();
long stargazersCount = doc["stargazers_count"];
Serial.println(stargazersCount);
delay(5000);
}
Build project
Run the following command to build project and upload program to the ESP8266 NodeMCU:
pio run --target upload
Testing
Open the Serial Monitor to see the stargazers count of GitHub repository.

Leave a Comment
Cancel reply