While working with ESP8266, one of the ways to debug code is to print data to the serial monitor when ESP8266 is connected via USB to a PC. However, there might be a cases when we want to access serial monitor remotely.
WebSerial is a library that provides web-based serial monitor for ESP8266 and ESP32 modules. Serial monitor can be accessed remotely using the IP Address of the module.
This tutorial provides example how to use remote serial monitor with WebSerial for ESP8266 NodeMCU development board.
Components
No. | Component | Quantity |
---|---|---|
1. | ESP8266 NodeMCU + Micro USB cable | 1 |
Library
WebSerial library and dependencies can be installed using PlatformIO from the command line.
pio project init --board nodemcuv2
pio lib install "me-no-dev/ESP Async WebServer"
pio lib install "ayushsharma82/WebSerial"
Project configuration file:
platformio.ini
[env:nodemcuv2]
platform = espressif8266
board = nodemcuv2
framework = arduino
lib_deps =
me-no-dev/ESP Async WebServer@^1.2
ayushsharma82/WebSerial@^1.1
Code
WebSerial is based on WebSockets. So we create an instance of AsyncWebServer
class that allows to run async HTTP and WebSocket server.
We define a callback function that will be executed when data is received from remote serial monitor.
In the setup
function we initialize serial communication and establish connection to a Wifi network. We print IP address of the ESP8266 to the serial port. We register a callback function with msgCallback
method. Server is started with begin
method.
In the loop
function we generate random number from 1 to 100 (inclusive) and send it to remote serial monitor.
src/main.cpp
#include <Arduino.h>
#include <ESP8266WiFi.h>
#include <ESPAsyncWebServer.h>
#include <WebSerial.h>
const char *WIFI_SSID = "YOUR WIFI NETWORK NAME";
const char *WIFI_PASSWORD = "YOUR WIFI PASSWORD";
AsyncWebServer server(80);
void callback(unsigned char* data, unsigned int length)
{
data[length] = '\0';
Serial.println((char*) data);
}
void setup()
{
Serial.begin(9600);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println(WiFi.localIP());
WebSerial.begin(&server);
WebSerial.msgCallback(callback);
server.begin();
}
void loop()
{
WebSerial.println((int) random(1, 101));
delay(1000);
}
Build project
Run the following command to build project and upload program to the ESP8266 NodeMCU:
pio run --target upload
Testing
Open a browser and access the remote serial monitor using http://<IP_ADDRESS>/webserial
URL, where <IP_ADDRESS>
is IP address of ESP8266 NodeMCU.
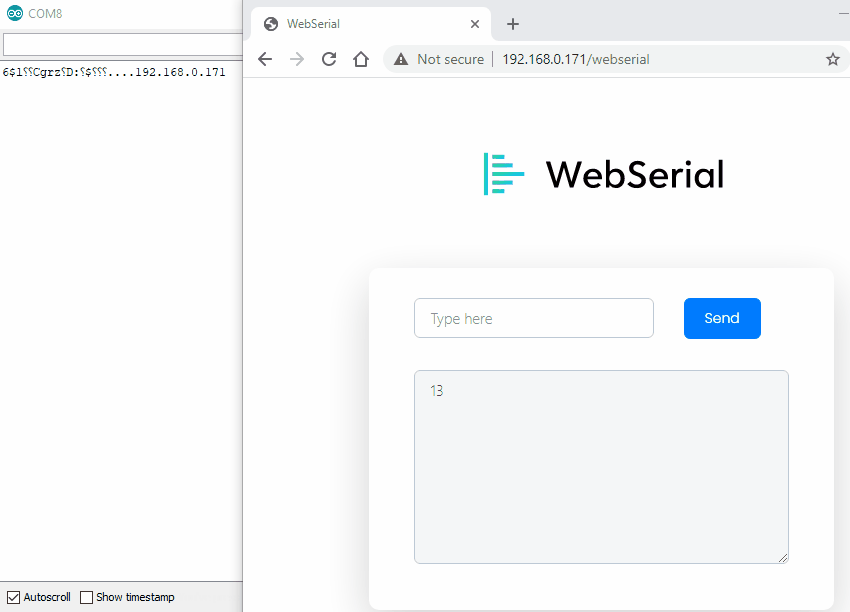
Leave a Comment
Cancel reply