The ESP8266 is a chip that has a full TCP/IP protocol stack. The ESP8266 can act as a MQTT client and subscribe messages from the MQTT broker for specified topic.
This tutorial provides example how to subscribe messages from the MQTT broker using ESP8266 NodeMCU development board.
Components
No. | Component | Quantity |
---|---|---|
1. | ESP8266 NodeMCU + Micro USB cable | 1 |
2. | MQTT broker. For example, Mosquitto running on Raspberry Pi | 1 |
Library
PubSubClient is an MQTT client library that allows to publish messages to a MQTT broker and subscribe to message topics. PubSubClient library can be installed using PlatformIO from the command line.
pio project init --board nodemcuv2
pio lib install "knolleary/PubSubClient"
Project configuration file:
platformio.ini
[env:nodemcuv2]
platform = espressif8266
board = nodemcuv2
framework = arduino
lib_deps = knolleary/PubSubClient@^2.8
Code
A Wi-Fi network SSID and password are defined as a constants. We also define constants to store connection details of the MQTT broker. It is the IP address or hostname of MQTT broker, the network port, the username and the corresponding password. We define a client ID that is used by MQTT broker to identify client. A client ID should be unique.
We will subscribe messages to a nodemcu/test
topic. In order to subscribe messages from the MQTT broker we create an instance of PubSubClient
class. We define a callback function that will be executed when a MQTT message is received. Bytes are converted into an integer and printed to the serial port.
In the setup
function serial communication is initialized and connection is established to a Wifi network. The hostname and the port of the MQTT broker are specified with setServer
method. We use the setCallback
method to register a callback function. We check whether the client is connected to the MQTT broker with connected
method. A client tries to connect to the MQTT broker by using connect
method. We do a while
loop until connection is successfully established. We use subscribe
method to subscribe messages to a nodemcu/test
topic.
In the loop
function we call the loop
method. It should be called regularly to allow the client maintain its connection to the MQTT broker and process subscribed messages.
src/main.cpp
#include <Arduino.h>
#include <ESP8266WiFi.h>
#include <PubSubClient.h>
const char *WIFI_SSID = "YOUR WIFI NETWORK NAME";
const char *WIFI_PASSWORD = "YOUR WIFI PASSWORD";
const char *MQTT_HOST = "192.168.0.184";
const int MQTT_PORT = 1883;
const char *MQTT_CLIENT_ID = "ESP8266 NodeMCU";
const char *MQTT_USER = "YOUR MQTT USER";
const char *MQTT_PASSWORD = "YOUR MQTT USER PASSWORD";
const char *TOPIC = "nodemcu/test";
WiFiClient client;
PubSubClient mqttClient(client);
void callback(char* topic, byte* payload, unsigned int length)
{
payload[length] = '\0';
int value = String((char*) payload).toInt();
Serial.println(topic);
Serial.println(value);
}
void setup()
{
Serial.begin(9600);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("Connected to Wi-Fi");
mqttClient.setServer(MQTT_HOST, MQTT_PORT);
mqttClient.setCallback(callback);
while (!client.connected()) {
if (mqttClient.connect(MQTT_CLIENT_ID, MQTT_USER, MQTT_PASSWORD )) {
Serial.println("Connected to MQTT broker");
} else {
delay(500);
Serial.print(".");
}
}
mqttClient.subscribe(TOPIC);
}
void loop()
{
mqttClient.loop();
}
Build project
Run the following command to build project and upload program to the ESP8266 NodeMCU:
pio run --target upload
Testing
We can test using MQTT Explorer. We publish messages on the nodemcu/test
topic. A topic and received messages are printed on the Serial Monitor.
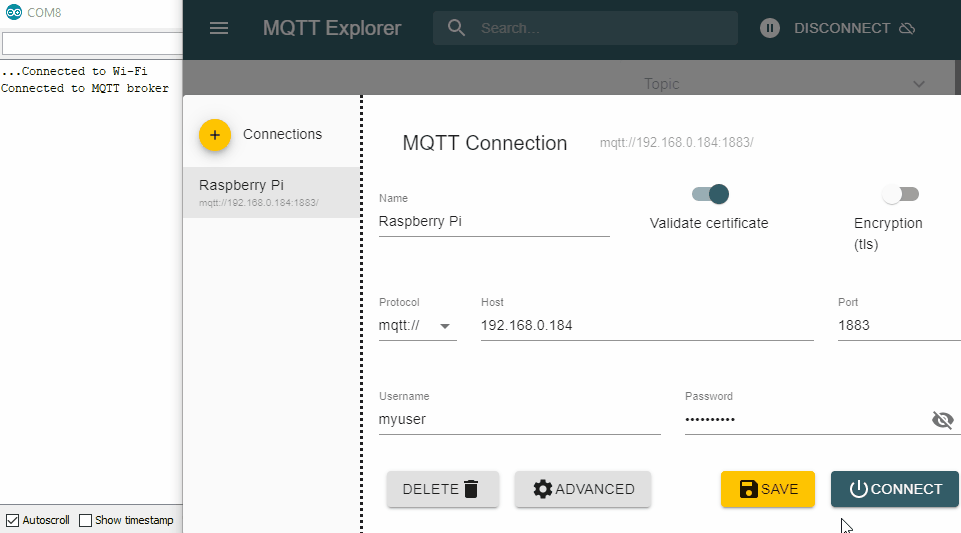
Leave a Comment
Cancel reply