Qt is a powerful cross-platform framework widely used for developing graphical user interfaces. While Qt provides a set of default styles for its widgets, you might want to customize the look and feel to match popular design frameworks like Bootstrap. This tutorial demonstrates how to make Qt 6 line edit to look in Bootstrap style.
The presented code aims to apply Bootstrap-inspired styling to Qt line edit widgets. Within the embedded CSS-like styling, various attributes are defined, including padding, border characteristics, text color, and font size.
The main function initializes a Qt application, incorporates the predefined style sheet, establishes a widget with a vertical layout, and adds two line edit widgets. Executing this code will result in a graphical user interface featuring Qt line edit widgets that mirror the Bootstrap design aesthetic.
#include <QApplication>
#include <QLineEdit>
#include <QVBoxLayout>
QString style = R"(
QLineEdit {
padding: 6px;
border: 1px solid #ccc;
border-radius: 4px;
color: #333;
font-size: 14px;
}
QLineEdit:focus {
border-color: #66afe9;
}
)";
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
app.setStyleSheet(style);
auto *window = new QWidget();
auto *layout = new QVBoxLayout();
window->setLayout(layout);
auto *lineEdit1 = new QLineEdit();
lineEdit1->setPlaceholderText("Email address");
layout->addWidget(lineEdit1);
auto *lineEdit2 = new QLineEdit();
lineEdit2->setPlaceholderText("Password");
lineEdit2->setEchoMode(QLineEdit::Password);
layout->addWidget(lineEdit2);
window->show();
return QApplication::exec();
}
Here's how the line edit appear:
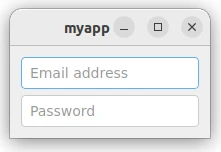
Leave a Comment
Cancel reply