An ultrasonic sensor HC-SR04 is an electronic device that allows to measure a distance. This sensor provides the non-contact distance measurement between 2 cm to 400 cm (1 in to 13 ft). Accuracy is about 3 mm (0.1 in).
The sensor has 4 pins: VCC (Power), TRIG (Trigger), ECHO (Receive), and GND (Ground). TRIG pin is used to trigger the sensor to sent out a high-frequency sound waves. The waves bounce off any nearby objects. Some of reflected waves comes back to the sensor. ECHO pin is used to generate a pulse when the reflected waves were received. The duration of the pulse can be used to calculate the distance to the object.
Components
No. | Component | Quantity |
---|---|---|
1. | Arduino Uno + USB cable | 1 |
2. | Ultrasonic sensor HC-SR04 | 1 |
3. | Wires | 4 |
Breadboard view
An ultrasonic sensor's ECHO pin is connected to the D2 pin on the Arduino Uno board, and the TRIG pin is connected to the D3 pin.
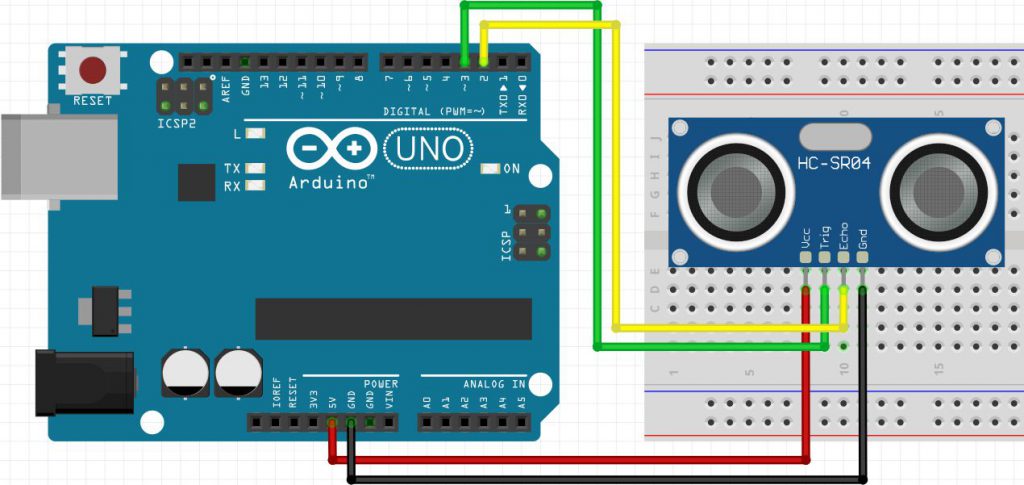
The table shows the connections.
No. | Arduino | HC-SR04 |
---|---|---|
1. | 5 V | VCC |
2. | GND | GND |
3. | D2 | ECHO |
4. | D3 | TRIG |
Schematic view
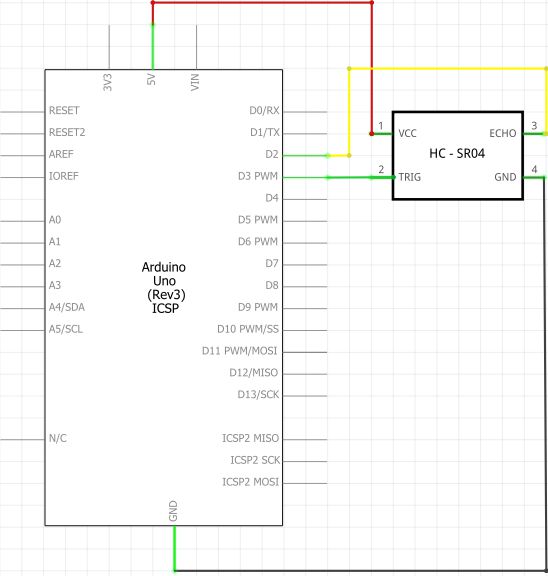
Code
First, ECHO and TRIG pins are defined. In the setup
function, we initialize serial communication at a baud rate of 9600. We set the ECHO pin as an input and the TRIG pin as an output.
To ensure a clean signal, we set LOW pulse for 2 microseconds (µs). We trigger a sensor by a HIGH pulse of 10 microseconds. To read the signal from the sensor, we use pulseIn
function. It returns the sound wave travel time in microseconds. We convert the duration to a distance. The speed of sound in air is about 340 m/s (0.034 cm/µs). Sound waves travels from the sensor to the object and comes back. So we divide the result by 2 because we need to find half of the travelled distance.
Finally, we print calculated distance in the serial monitor.
#define ECHO_PIN 2
#define TRIG_PIN 3
void setup() {
Serial.begin(9600);
pinMode(ECHO_PIN, INPUT);
pinMode(TRIG_PIN, OUTPUT);
}
void loop() {
int distance = readDistance();
Serial.print(distance);
Serial.println(" cm");
delay(5);
}
int readDistance() {
digitalWrite(TRIG_PIN, LOW);
delayMicroseconds(2);
digitalWrite(TRIG_PIN, HIGH);
delayMicroseconds(10);
digitalWrite(TRIG_PIN, LOW);
long duration = pulseIn(ECHO_PIN, HIGH);
return duration * 0.034 / 2;
}
Leave a Comment
Cancel reply