There are various algorithms that allow to reduce noise in an image. One of them is the median filter. This filter calculates the median of all the pixels in the kernel area. Then the value of the central pixel is replaced by the calculated median. Median filter is widely used to remove "salt and pepper" type noise.
OpenCV offers the medianBlur
function to apply a median filter to an image. This function accepts kernel size. It should be an odd integer greater than 1 (e.g. 3, 5, 7, …).
import cv2
img = cv2.imread('test.jpg')
grayImg = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
filteredImg = cv2.medianBlur(grayImg, ksize=5)
cv2.imshow('Original image', grayImg)
cv2.imshow('Filtered image', filteredImg)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat img = imread("test.jpg");
Mat grayImg;
cvtColor(img, grayImg, COLOR_BGR2GRAY);
Mat filteredImg;
medianBlur(grayImg, filteredImg, 5);
imshow("Original image", grayImg);
imshow("Filtered image", filteredImg);
waitKey(0);
destroyAllWindows();
return 0;
}
#include <opencv2/opencv.hpp>
#include <opencv2/cudaimgproc.hpp>
#include <opencv2/cudafilters.hpp>
using namespace cv;
int main()
{
Mat img = imread("test.jpg");
cuda::GpuMat gpuImg(img);
cuda::cvtColor(gpuImg, gpuImg, COLOR_RGB2GRAY);
Mat grayImg(gpuImg);
Ptr<cuda::Filter> filter = cuda::createMedianFilter(gpuImg.type(), 5);
filter->apply(gpuImg, gpuImg);
Mat filteredImg(gpuImg);
imshow("Original image", grayImg);
imshow("Filtered image", filteredImg);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat img = Imgcodecs.imread("test.jpg");
Mat grayImg = new Mat();
Imgproc.cvtColor(img, grayImg, Imgproc.COLOR_BGR2GRAY);
Mat filteredImg = new Mat();
Imgproc.medianBlur(grayImg, filteredImg, 5);
HighGui.imshow("Original image", grayImg);
HighGui.imshow("Filtered image", filteredImg);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
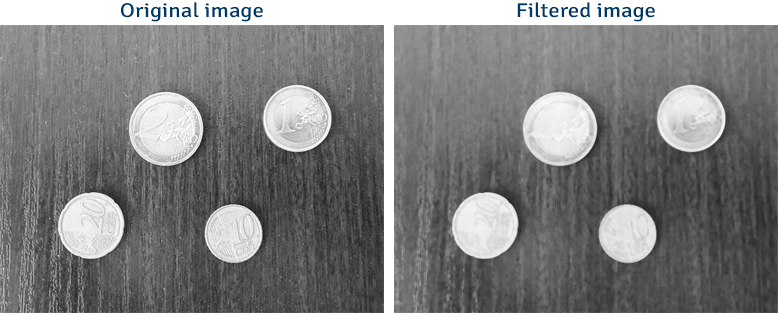
Leave a Comment
Cancel reply