Qt is a powerful cross-platform application framework that allows developers to create user interfaces and applications with ease. One common task in software development is accessing and displaying environment variables. Environment variables hold crucial information about the system environment and are often used in applications for configuration. This tutorial demonstrates how to display environment variables in a table using Qt 6.
The following code creates a simple application that displays environment variables in a table. It sets up a window with a table widget having two columns labeled "Key" and "Value". Then, it retrieves system environment variables using QProcessEnvironment
, iterates through them, and adds each variable and its corresponding value to the table.
#include <QApplication>
#include <QProcessEnvironment>
#include <QTableWidget>
#include <QHeaderView>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QTableWidget table;
table.setColumnCount(2);
table.setHorizontalHeaderLabels({"Key", "Value"});
table.horizontalHeader()->setSectionResizeMode(QHeaderView::Stretch);
QProcessEnvironment env = QProcessEnvironment::systemEnvironment();
for (const QString &key: env.keys()) {
int row = table.rowCount();
table.insertRow(row);
table.setItem(row, 0, new QTableWidgetItem(key));
table.setItem(row, 1, new QTableWidgetItem(env.value(key)));
}
table.resize(800, 600);
table.show();
return QApplication::exec();
}
Here's how the application might look:
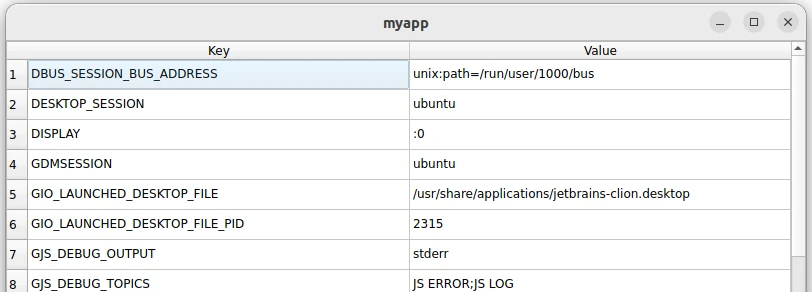
Leave a Comment
Cancel reply