When working with C++ projects, managing dependencies is an important aspect of development. One popular tool for managing C++ library dependencies is vcpkg. However, keeping track of installed packages can become cumbersome. This tutorial shows how to display installed vcpkg packages in table using Qt 6.
Prepare environment
Before starting, make sure that vcpkg
is installed on the system.
Code
The provided code snippet utilizes the Qt framework to create a graphical application that displays information about installed vcpkg packages in a table format. Upon execution, the application creates a table widget with three columns. It then invokes a sub-process to execute the vcpkg list
command with the --x-json
option to retrieve package information in JSON format.
After parsing the JSON output, the code iterates over each package, extracting its name, version, and features. These details are then inserted into the table widget as individual rows. Finally, the application sets the size of the table, and displays it.
#include <QApplication>
#include <QTableWidget>
#include <QHeaderView>
#include <QProcess>
#include <QJsonDocument>
#include <QJsonObject>
#include <QJsonArray>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QTableWidget table;
table.setColumnCount(3);
table.setHorizontalHeaderLabels({"Package", "Version", "Features"});
table.horizontalHeader()->setSectionResizeMode(QHeaderView::Stretch);
table.verticalHeader()->setSectionResizeMode(QHeaderView::ResizeToContents);
QProcess proc;
proc.start("vcpkg", QStringList() << "list" << "--x-json");
proc.waitForFinished();
QJsonObject json = QJsonDocument::fromJson(proc.readAllStandardOutput()).object();
for (const QString &key: json.keys()) {
QJsonValue value = json.value(key);
QString features;
for (const QJsonValueRef &feature: value["features"].toArray()) {
features += feature.toString() + ", ";
}
features.chop(2);
int row = table.rowCount();
table.insertRow(row);
table.setItem(row, 0, new QTableWidgetItem(key));
table.setItem(row, 1, new QTableWidgetItem(value["version"].toString()));
table.setItem(row, 2, new QTableWidgetItem(features));
}
table.resize(800, 600);
table.show();
return QApplication::exec();
}
Here's how the application might look:
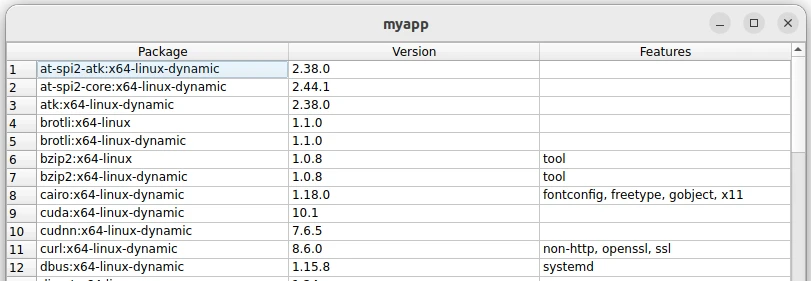
Leave a Comment
Cancel reply