A floating action button (FAB) is a circular button that contains an icon in the center. FAB is used to perform the primary action on a screen. Recommend using a single FAB per screen and for positive actions such as "add", "send", "call", etc.
Firstly, we need to add the Material Components dependency in the module's build.gradle
file.
app/build.gradle
dependencies {
// Other dependencies
// ...
implementation 'com.google.android.material:material:1.2.0'
}
Now we can add the FloatingActionButton
in the layout XML file.
By default, the background color of the FAB is represented by the theme's accent color. This color is defined by the colorAccent
attribute in the res/values/styles.xml
file. We can use the android:backgroundTint
attribute to change the background color of the FAB.
The FAB icon is set by using the android:src
attribute. The color of the icon can be changed with android:tint
attribute.
The app:fabSize
attribute is used to define the size of the FAB.
app/src/main/res/layout/activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.coordinatorlayout.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/myButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_margin="16dp"
android:backgroundTint="#4287f5"
android:src="@drawable/ic_add"
android:tint="#ffffff"
app:fabSize="normal" />
</androidx.coordinatorlayout.widget.CoordinatorLayout>
We can use setOnClickListener()
method to handle FAB clicks.
app/src/main/java/com/example/app/MainActivity.kt
package com.example.app
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.util.Log
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity()
{
override fun onCreate(savedInstanceState: Bundle?)
{
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
myButton.setOnClickListener {
Log.i("MY_APP", "Clicked")
}
}
}
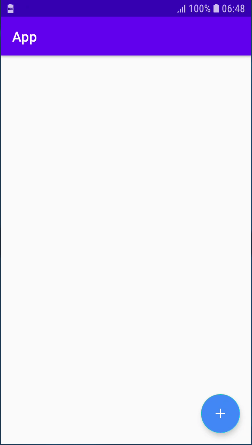
Leave a Comment
Cancel reply