The options menu is the main collection of menu items associated with an activity. In this menu, we should place actions that have a global impact on the application. For example, "Account", "Settings", "Help", etc.
First, we need to create a new menu
folder in resource directory (res/menu/
). To define the options menu, we need to create an XML file in the res/menu/
directory.
The <menu>
element is a container for menu items. The <item>
element is used to define an item in a menu. We can create a submenu by adding a <menu>
element as the child of an <item>
.
By using the app:showAsAction
attribute, we can define how the item should appear as an action item in the app bar. The ifRoom
value means that the item appears in the app bar only if there is sufficient space in the app bar.
app/src/main/res/menu/main_menu.xml
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<item
android:id="@+id/search"
android:icon="@drawable/ic_search"
android:title="Search"
app:showAsAction="ifRoom" />
<item
android:id="@+id/account"
android:title="Account"
app:showAsAction="never" />
<item
android:title="Settings"
app:showAsAction="never">
<menu>
<item
android:id="@+id/storage"
android:title="Storage" />
<item
android:id="@+id/sound"
android:title="Sound" />
</menu>
</item>
</menu>
To load the options menu for an activity, we need to override onCreateOptionsMenu()
method. After that, we can load a menu resource defined in an XML file by using MenuInflater.inflate()
method.
The system invokes the onOptionsItemSelected()
method when the user selects an item from the options menu. The method has the MenuItem
parameter which defines the selected item.
app/src/main/java/com/example/app/MainActivity.kt
package com.example.app
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.view.Menu
import android.view.MenuItem
import android.widget.Toast
class MainActivity : AppCompatActivity()
{
override fun onCreate(savedInstanceState: Bundle?)
{
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
override fun onCreateOptionsMenu(menu: Menu): Boolean
{
menuInflater.inflate(R.menu.main_menu, menu)
return true
}
override fun onOptionsItemSelected(item: MenuItem): Boolean
{
return when (item.itemId) {
R.id.search -> {
Toast.makeText(this, "Search", Toast.LENGTH_SHORT).show()
true
}
R.id.account -> {
Toast.makeText(this, "Account", Toast.LENGTH_SHORT).show()
true
}
R.id.storage -> {
Toast.makeText(this, "Storage", Toast.LENGTH_SHORT).show()
true
}
R.id.sound -> {
Toast.makeText(this, "Sound", Toast.LENGTH_SHORT).show()
true
}
else -> super.onOptionsItemSelected(item)
}
}
}
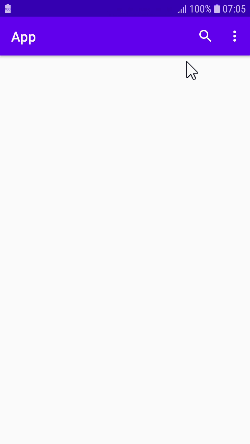
Leave a Comment
Cancel reply