Material Components library allows displaying a shapeable image. By setting corner radius and family we are able to change the shape of the image to circle, rhombus, octagon, etc.
Firstly, we need to add the Material Components dependency in the module's build.gradle
file.
app/build.gradle
dependencies {
// Other dependencies
// ...
implementation 'com.google.android.material:material:1.2.0'
}
A shape of the image is declared in a style resource file.
app/src/main/res/values/styles.xml
<resources>
<!-- Other styles ... -->
<style name="ImageViewCircle" parent="">
<item name="cornerFamily">rounded</item>
<item name="cornerSize">50%</item>
</style>
<style name="ImageViewCornerCut" parent="">
<item name="cornerFamily">cut</item>
<item name="cornerSize">20%</item>
</style>
</resources>
Now we can add the ShapeableImageView
in the layout XML file. The app:shapeAppearanceOverlay
attribute defines a style reference that contains shape values.
By using the cornerFamily
attribute, we can set the type of corners: rounded or cut. The cornerFamily
attribute affects all four corners, but we can modify individuals corners as well (e.g. cornerFamilyTopLeft
).
The size of corners can be set with cornerSize
attribute. We can use corresponding attributes to modify individual corners (e.g. cornerSizeTopLeft
).
We can define the stroke color and stroke width by using app:strokeColor
and app:strokeWidth
attributes.
app/src/main/res/layout/activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<com.google.android.material.imageview.ShapeableImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="4dp"
android:src="@drawable/orange"
app:shapeAppearanceOverlay="@style/ImageViewCircle"
app:strokeColor="#00BCD4"
app:strokeWidth="4dp" />
<com.google.android.material.imageview.ShapeableImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/orange"
app:shapeAppearanceOverlay="@style/ImageViewCornerCut" />
</LinearLayout>
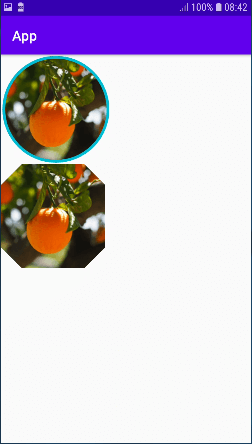
Leave a Comment
Cancel reply