In modern desktop applications, it's becoming increasingly common to work with multiple monitors, offering users expanded workspace and improved productivity. However, managing and utilizing these additional screens effectively often poses a challenge for developers. In Qt, one of the challenges developers face is displaying information about each monitor to users. This tutorial shows how to display information for each monitor in Qt 6.
The provided code displays information about each screen connected to the system. It begins by initializing a Qt application and creating a main window widget. For each screen, the code creates a group box to contain the screen information, then adds vertically arranged labels displaying details such as the screen model, serial number, resolution, refresh rate, and color depth. Finally, it adds each group box to the main layout of the window and displays the window to the user.
#include <QApplication>
#include <QHBoxLayout>
#include <QGroupBox>
#include <QLabel>
#include <QScreen>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
auto *window = new QWidget();
QHBoxLayout layout(window);
for (auto screen : QApplication::screens()) {
auto *groupBox = new QGroupBox(window);
auto *vlayout = new QVBoxLayout(groupBox);
vlayout->addWidget(new QLabel("Model: " + screen->model()));
vlayout->addWidget(new QLabel("Serial number: " + screen->serialNumber()));
auto width = QString::number(screen->size().width());
auto height = QString::number(screen->size().height());
vlayout->addWidget(new QLabel("Resolution: " + width + " x " + height));
auto refreshRate = QString::number(screen->refreshRate());
vlayout->addWidget(new QLabel("Refresh rate: " + refreshRate + " Hz"));
auto depth = QString::number(screen->depth());
vlayout->addWidget(new QLabel("Color depth: " + depth + " bit"));
layout.addWidget(groupBox);
}
window->show();
return QApplication::exec();
}
Here's how the application might look:
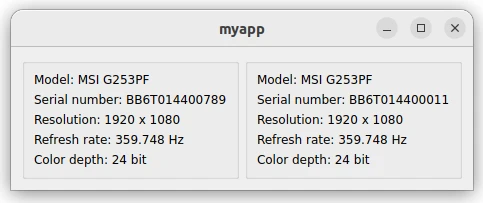
Leave a Comment
Cancel reply