When developing applications, it's often necessary to retrieve and display system information for various purposes such as troubleshooting, or simply providing users with insight into their system's configuration. Various operating systems utilize different APIs and mechanisms for retrieving system information. Qt simplifies complex tasks by providing solutions that hide much of the complexity. This tutorial demonstrates how to display system information in Qt 6.
The provided code creates a simple Qt application that displays various system information. It first initializes the application and creates a new window. Inside the window, a vertical layout is set up. Then, several label widgets are added to the layout, each containing a specific piece of system information retrieved using QSysInfo
functions. This includes the hostname, CPU architecture, kernel version, kernel type, product name, product type, and product version.
#include <QApplication>
#include <QVBoxLayout>
#include <QLabel>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
auto *window = new QWidget();
QVBoxLayout layout(window);
layout.addWidget(new QLabel("Hostname: " + QSysInfo::machineHostName()));
layout.addWidget(new QLabel("CPU architecture: " + QSysInfo::currentCpuArchitecture()));
layout.addWidget(new QLabel("Kernel version: " + QSysInfo::kernelVersion()));
layout.addWidget(new QLabel("Kernel type: " + QSysInfo::kernelType()));
layout.addWidget(new QLabel("Product name: " + QSysInfo::prettyProductName()));
layout.addWidget(new QLabel("Product type: " + QSysInfo::productType()));
layout.addWidget(new QLabel("Product version: " + QSysInfo::productVersion()));
window->show();
return QApplication::exec();
}
Here's how the application might look:
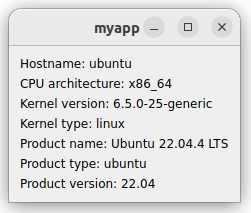
Leave a Comment
Cancel reply