A MediaPlayer allows playing audio in Android application. Audio can be played from various sources, such as app's resources, external storage, from the internet.
In the layout XML file, we added two Button
elements that will be used to control audio playback.
app/src/main/res/layout/activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<Button
android:id="@+id/startButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Start" />
<Button
android:id="@+id/stopButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Stop" />
</LinearLayout>
Under the res
directory, create a new raw
resource directory. Put the audio file into the raw
directory.
app/src/main/res/raw/audio.mp3
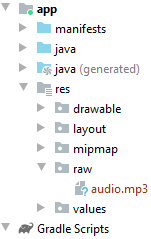
We created an instance of MediaPlayer
and set the URI of the audio.
The player for playback is prepared by using the prepare()
method. When the application is stopped, we release resources associated with an instance of MediaPlayer
by using release()
method.
app/src/main/java/com/example/app/MainActivity.kt
package com.example.app
import android.media.MediaPlayer
import android.net.Uri
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity()
{
private lateinit var mediaPlayer: MediaPlayer
override fun onCreate(savedInstanceState: Bundle?)
{
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val path = "android.resource://" + packageName + "/" + R.raw.audio
mediaPlayer = MediaPlayer()
mediaPlayer.setDataSource(this, Uri.parse(path))
startButton.setOnClickListener {
if (!mediaPlayer.isPlaying) {
mediaPlayer.prepare()
mediaPlayer.start()
}
}
stopButton.setOnClickListener {
if (mediaPlayer.isPlaying) {
mediaPlayer.stop()
}
}
}
override fun onStop()
{
mediaPlayer.release()
super.onStop()
}
}
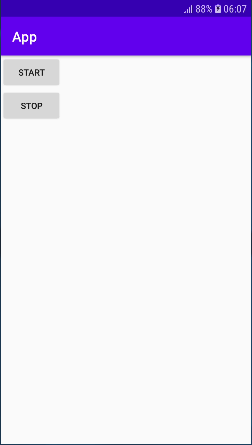
Leave a Comment
Cancel reply