A VideoView allows playing video in Android application. Video can be played from various sources, such as app's resources, external storage, from the internet.
In the layout XML file we added VideoView
which provides a surface to play a video.
app/src/main/res/layout/activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<VideoView
android:id="@+id/myVideoView"
android:layout_width="wrap_content"
android:layout_height="200dp"
android:layout_centerInParent="true" />
</RelativeLayout>
Under the res
directory, create a new raw
resource directory. Put the video file into the raw
directory.
app/src/main/res/raw/video.mp4
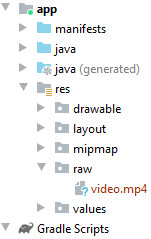
The MediaController
class provides controls that allow to manage a video playback. It contains the buttons like "Play/Pause", "Rewind", and "Fast Forward". An instance of the MediaController
is attached to VideoView
.
We set the URI of the video to VideoView
and then start the playback.
app/src/main/java/com/example/app/MainActivity.kt
package com.example.app
import android.net.Uri
import android.os.Bundle
import android.widget.MediaController
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity()
{
override fun onCreate(savedInstanceState: Bundle?)
{
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val path = "android.resource://" + packageName + "/" + R.raw.video
val mediaController = MediaController(this)
mediaController.setAnchorView(myVideoView)
myVideoView.setMediaController(mediaController)
myVideoView.setVideoURI(Uri.parse(path))
myVideoView.start()
}
}
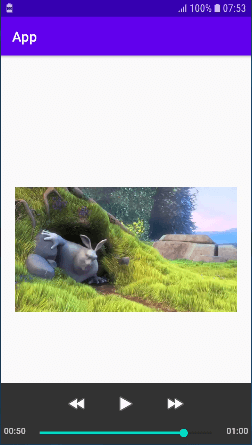
Leave a Comment
Cancel reply