There are various ways to authenticate an API user in Laravel application. API authentication can be implemented using Laravel Passport, Laravel Sanctum, etc. External packages offer great features, but there might be cases when we need a simple API authentication that can be implemented using Laravel built-in authentication services without installing additional packages.
This tutorial shows how to implement simple token-based API authentication in Laravel application.
First, we need to create a migration that adds the api_token
column in the users
table.
php artisan make:migration add_api_token_to_users_table
We defined a unique api_token
column that length is 32 characters. Note that you can choose a different column length.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class AddApiTokenToUsersTable extends Migration
{
public function up(): void
{
Schema::table('users', function (Blueprint $table) {
$table->char('api_token', 32)->unique()->nullable()->after('password');
});
}
public function down(): void
{
Schema::table('users', function (Blueprint $table) {
$table->dropColumn('api_token');
});
}
}
Run migration by executing the following command:
php artisan migrate
For testing, we can execute the following SQL query to add a user in the table:
INSERT INTO users (name, email, password, api_token, remember_token, created_at, updated_at)
VALUES ('Tester',
'tester@example.com',
'$2y$10$92IXUNpkjO0rOQ5byMi.Ye4oKoEa3Ro9llC/.og/at2.uheWG/igi',
'G2iIJV3qIoXg92nuGwu2WKbAv6jTExiO',
'AQ7gRgu0iQ',
'2021-01-01 12:00:54',
'2021-01-01 12:00:54');
By default, an api
guard is already defined, and it uses token
authentication driver and users
table where stored API tokens. So make sure that your config/auth.php
configuration file has an api
guard defined as follows:
config/auth.php
<?php
return [
// ...
'guards' => [
'web' => [
'driver' => 'session',
'provider' => 'users',
],
'api' => [
'driver' => 'token',
'provider' => 'users',
'hash' => false,
],
],
// ...
];
Now we can specify the auth:api
middleware on routes that needs token-based API authentication. API routes defined in routes/api.php
file.
routes/api.php
<?php
use Illuminate\Http\JsonResponse;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
Route::middleware('auth:api')->post('/test', function (Request $request) {
return new JsonResponse(['message' => 'Hello world']);
});
To test an API authentication we can use Postman tool which can be downloaded in the official website.
There are various ways to provide an API token to Laravel application:
- API token can be provided as a query string with
api_token
key.
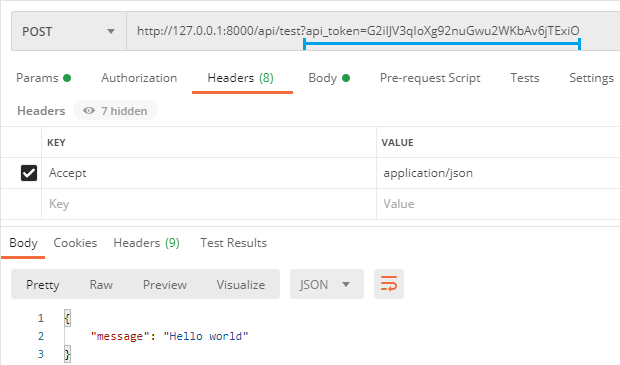
- An API token can be provided in the request body with the
api_token
key.
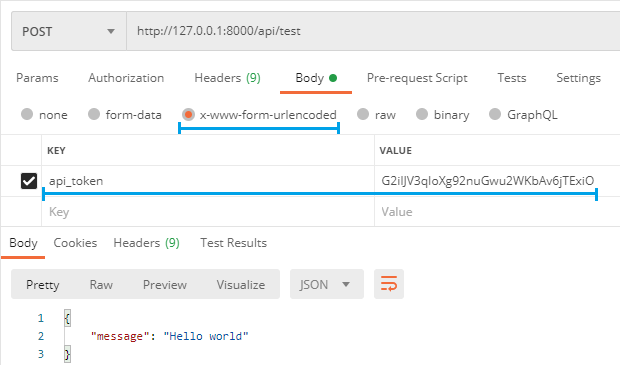
- API token can be provided as a
Bearer
token in theAuthorization
header.
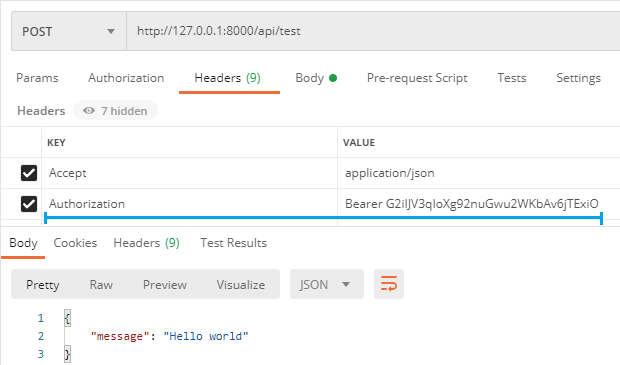
Note that we must to include Accept: application/json
header in the request to get JSON as a response if a user not authenticated.
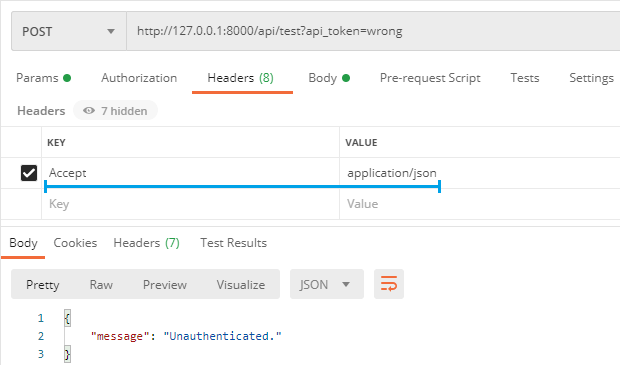
Leave a Comment
Cancel reply