Pulse width modulation (PWM) is a technique that allows to control analog devices with a digital signal. Raspberry Pi Pico has GPIO pins that can be configured as PWM signal output. This tutorial provides circuit diagram and code example how to control an LED brightness with PWM and Raspberry Pi Pico.
Components
No. | Component | Quantity |
---|---|---|
1. | Raspberry Pi Pico | 1 |
2. | 330Ω resistor | 1 |
3. | LED | 1 |
Circuit diagram
LED is connected to resistor and it connected to pin 15 (GP15) on Raspberry Pi Pico. GP15 can be configured as PWM output.
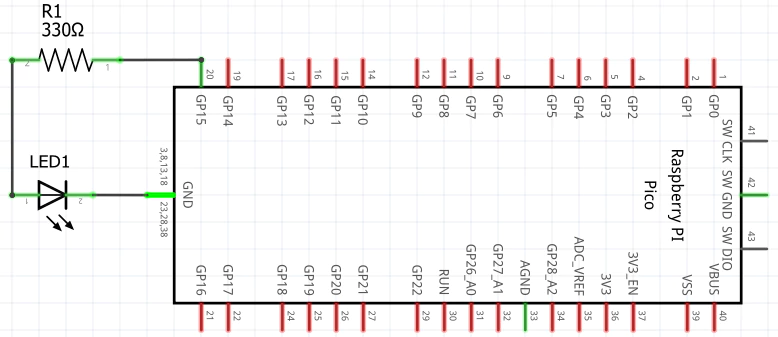
Code
Using gpio_set_function
function, Pin 15 is configured as PWM output. The pwm_get_default_config
function returns default values for PWM configuration. That is used to initialize PWM with pwm_init
function. Inside the infinite while loop, we set PWM output level from 0 to 65535 using pwm_set_gpio_level
function which allows to control an LED brightness. PWM output level goes up then down. Level changes once per 50 microseconds.
project/main.c
#include <pico/stdlib.h>
#include <hardware/pwm.h>
int main()
{
const uint LED_PIN = 15;
const uint MAX_PWM_LEVEL = 65535;
gpio_set_function(LED_PIN, GPIO_FUNC_PWM);
uint sliceNum = pwm_gpio_to_slice_num(LED_PIN);
pwm_config config = pwm_get_default_config();
pwm_init(sliceNum, &config, true);
int level = 0;
bool up = true;
while (true) {
pwm_set_gpio_level(LED_PIN, level);
if (level == 0) {
up = true;
} else if (level == MAX_PWM_LEVEL) {
up = false;
}
level = up ? level + 1 : level - 1;
sleep_us(50);
}
}
In the CMakeLists.txt
add the hardware_pwm
library as dependency.
project/CMakeLists.txt
cmake_minimum_required(VERSION 3.13)
include($ENV{PICO_SDK_PATH}/external/pico_sdk_import.cmake)
project(myapp C CXX ASM)
set(CMAKE_C_STANDARD 11)
set(CMAKE_CXX_STANDARD 17)
pico_sdk_init()
add_executable(${PROJECT_NAME} main.c)
pico_add_extra_outputs(${PROJECT_NAME})
target_link_libraries(${PROJECT_NAME} pico_stdlib hardware_pwm)
Designed circuit
Project was built and binary file myapp.uf2
was moved to the mounted drive RPI-RP2. The brightness of LED is changed according to PWM output level. The higher PWM level is, the brighter LED is. The lower PWM level is, the darker LED is.
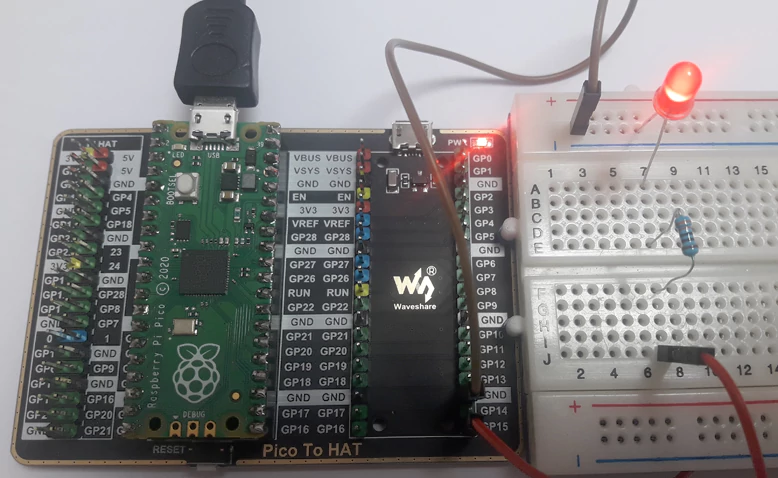
Leave a Comment
Cancel reply