For some applications, there might be a requirement to add a custom HTTP header to all the responses. It can be done by adding header on each controller method. However, a better solution is to add header globally to all the responses.
This tutorial provides example how to add custom header to every response in Laravel 9 application.
Create a new middleware and add a custom HTTP header to response.
app/Http/Middleware/AddHeadersToResponse.php
<?php
namespace App\Http\Middleware;
use Closure;
use Illuminate\Http\Request;
class AddHeadersToResponse
{
public function handle(Request $request, Closure $next): mixed
{
$response = $next($request);
$response->header('X-App-Message', 'Hello world');
return $response;
}
}
Define the middleware in the middleware
array which can be found in the Kernel.php
file.
app/Http/Kernel.php
<?php
namespace App\Http;
use App\Http\Middleware\AddHeadersToResponse;
use Illuminate\Foundation\Http\Kernel as HttpKernel;
class Kernel extends HttpKernel
{
protected $middleware = [
// ...
AddHeadersToResponse::class,
];
// ...
}
Response headers can be verified using web browser:
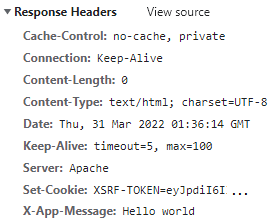
The 2 Comments Found
Hi, in which use cases this feature would be necessary?
Hi,
Most of the time, the problem can be solved in different way, but there are scenarios when this feature can be useful. Adding custom headers in HTTP responses can be useful for logging purposes. For example, X-RESPONSE-ID header can be logged in client side that consumes API. It can be useful to identify each HTTP response in the future. Also, custom headers can be used to add additional metadata to HTTP responses, such as cache control settings, or other information that can be useful for clients that consumes API.
Leave a Comment
Cancel reply