Closing is a morphological image processing operation when dilation operation is applied to an image and then erosion operation. Closing operation is useful to fill small holes in objects while preserving the size of large holes and objects.
The morphologyEx
function with MORPH_CLOSE
parameter can be used to apply the closing operation to an image.
import cv2
import numpy as np
inputImg = cv2.imread('test.jpg')
inputImg = cv2.cvtColor(inputImg, cv2.COLOR_BGR2GRAY)
_, inputImg = cv2.threshold(inputImg, 0, 255, cv2.THRESH_OTSU)
kernel = np.ones((4, 4))
outputImg = cv2.morphologyEx(inputImg, cv2.MORPH_CLOSE, kernel)
cv2.imshow('Input image', inputImg)
cv2.imshow('Output image', outputImg)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat inputImg = imread("test.jpg");
cvtColor(inputImg, inputImg, COLOR_BGR2GRAY);
threshold(inputImg, inputImg, 0, 255, THRESH_OTSU);
Mat kernel = getStructuringElement(MORPH_RECT, Size(4, 4));
Mat outputImg;
morphologyEx(inputImg, outputImg, MORPH_CLOSE, kernel);
imshow("Input image", inputImg);
imshow("Output image", outputImg);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.*;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat inputImg = Imgcodecs.imread("test.jpg");
Imgproc.cvtColor(inputImg, inputImg, Imgproc.COLOR_BGR2GRAY);
Imgproc.threshold(inputImg, inputImg, 0, 255, Imgproc.THRESH_OTSU);
Mat kernel = Imgproc.getStructuringElement(Imgproc.MORPH_RECT, new Size(4, 4));
Mat outputImg = new Mat();
Imgproc.morphologyEx(inputImg, outputImg, Imgproc.MORPH_CLOSE, kernel);
HighGui.imshow("Input image", inputImg);
HighGui.imshow("Output image", outputImg);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
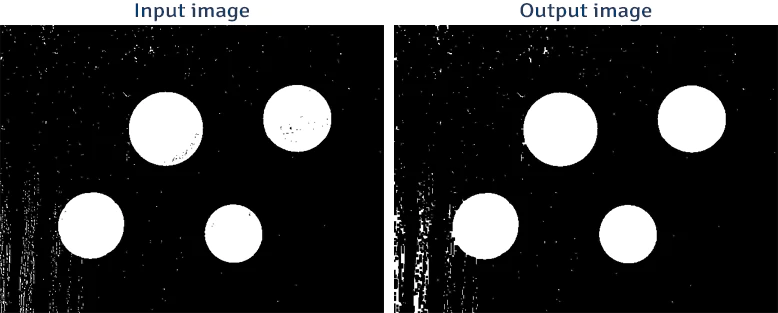
Leave a Comment
Cancel reply