Morphological gradient is an image processing operation. It is the difference between dilation and erosion operations. Morphological gradient can be useful to determine the outline of the object.
The morphologyEx
function with MORPH_GRADIENT
parameter can be used to apply the morphological gradient operation to an image.
import cv2
import numpy as np
inputImg = cv2.imread('test.jpg')
inputImg = cv2.cvtColor(inputImg, cv2.COLOR_BGR2GRAY)
_, inputImg = cv2.threshold(inputImg, 0, 255, cv2.THRESH_OTSU)
kernel = np.ones((4, 4))
outputImg = cv2.morphologyEx(inputImg, cv2.MORPH_GRADIENT, kernel)
cv2.imshow('Input image', inputImg)
cv2.imshow('Output image', outputImg)
cv2.waitKey(0)
cv2.destroyAllWindows()
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat inputImg = imread("test.jpg");
cvtColor(inputImg, inputImg, COLOR_BGR2GRAY);
threshold(inputImg, inputImg, 0, 255, THRESH_OTSU);
Mat kernel = getStructuringElement(MORPH_RECT, Size(4, 4));
Mat outputImg;
morphologyEx(inputImg, outputImg, MORPH_GRADIENT, kernel);
imshow("Input image", inputImg);
imshow("Output image", outputImg);
waitKey(0);
destroyAllWindows();
return 0;
}
package app;
import org.opencv.core.*;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
public class Main
{
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public static void main(String[] args)
{
Mat inputImg = Imgcodecs.imread("test.jpg");
Imgproc.cvtColor(inputImg, inputImg, Imgproc.COLOR_BGR2GRAY);
Imgproc.threshold(inputImg, inputImg, 0, 255, Imgproc.THRESH_OTSU);
Mat kernel = Imgproc.getStructuringElement(Imgproc.MORPH_RECT, new Size(4, 4));
Mat outputImg = new Mat();
Imgproc.morphologyEx(inputImg, outputImg, Imgproc.MORPH_GRADIENT, kernel);
HighGui.imshow("Input image", inputImg);
HighGui.imshow("Output image", outputImg);
HighGui.waitKey(0);
HighGui.destroyAllWindows();
System.exit(0);
}
}
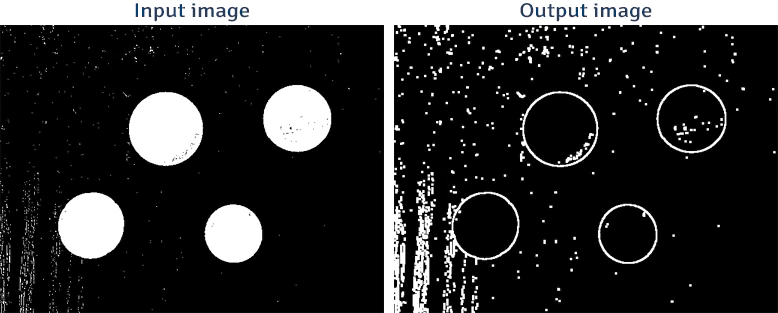
Leave a Comment
Cancel reply